biweekly-contest-77
A
Statement
Metadata
- Link: 统计是给定字符串前缀的字符串数目
- Difficulty: Easy
- Tag:
给你一个字符串数组 words
和一个字符串 s
,其中 words[i]
和 s
只包含 小写英文字母 。
请你返回 words
中是字符串 s
前缀 的 字符串数目 。
一个字符串的 前缀 是出现在字符串开头的子字符串。子字符串 是一个字符串中的连续一段字符序列。
示例 1:
输入:words = ["a","b","c","ab","bc","abc"], s = "abc"
输出:3
解释:
words 中是 s = "abc" 前缀的字符串为:
"a" ,"ab" 和 "abc" 。
所以 words 中是字符串 s 前缀的字符串数目为 3 。
示例 2:
输入:words = ["a","a"], s = "aa"
输出:2
解释:
两个字符串都是 s 的前缀。
注意,相同的字符串可能在 words 中出现多次,它们应该被计数多次。
提示:
1 <= words.length <= 1000
1 <= words[i].length, s.length <= 10
words[i]
和s
只 包含小写英文字母。
Metadata
- Link: Count Prefixes of a Given String
- Difficulty: Easy
- Tag:
You are given a string array words
and a string s
, where words[i]
and s
comprise only of lowercase English letters.
Return the number of strings in words
that are a prefix of s
.
A prefix of a string is a substring that occurs at the beginning of the string. A substring is a contiguous sequence of characters within a string.
Example 1:
Input: words = ["a","b","c","ab","bc","abc"], s = "abc"
Output: 3
Explanation:
The strings in words which are a prefix of s = "abc" are:
"a", "ab", and "abc".
Thus the number of strings in words which are a prefix of s is 3.
Example 2:
Input: words = ["a","a"], s = "aa"
Output: 2
Explanation:
Both of the strings are a prefix of s.
Note that the same string can occur multiple times in words, and it should be counted each time.
Constraints:
1 <= words.length <= 1000
1 <= words[i].length, s.length <= 10
words[i]
ands
consist of lowercase English letters only.
Solution
#include <bits/stdc++.h>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
#define endl "\n"
#define fi first
#define se second
#define all(x) begin(x), end(x)
#define rall rbegin(a), rend(a)
#define bitcnt(x) (__builtin_popcountll(x))
#define complete_unique(a) a.erase(unique(begin(a), end(a)), end(a))
#define mst(x, a) memset(x, a, sizeof(x))
#define MP make_pair
using ll = long long;
using ull = unsigned long long;
using db = double;
using ld = long double;
using VLL = std::vector<ll>;
using VI = std::vector<int>;
using PII = std::pair<int, int>;
using PLL = std::pair<ll, ll>;
using namespace __gnu_pbds;
using namespace std;
template <typename T>
using ordered_set = tree<T, null_type, less<T>, rb_tree_tag, tree_order_statistics_node_update>;
template <typename T, typename S>
inline bool chmax(T &a, const S &b) {
return a < b ? a = b, 1 : 0;
}
template <typename T, typename S>
inline bool chmin(T &a, const S &b) {
return a > b ? a = b, 1 : 0;
}
#ifdef LOCAL
#include <debug.hpp>
#else
#define dbg(...)
#endif
// head
class Solution {
public:
int countPrefixes(vector<string> &words, string s) {
int res = 0;
for (const auto &w : words) {
if (w.length() <= s.length() && s.substr(0, w.length()) == w) {
++res;
}
}
return res;
}
};
#ifdef LOCAL
int main() {
return 0;
}
#endif
B
Statement
Metadata
- Link: 最小平均差
- Difficulty: Medium
- Tag:
给你一个下标从 0 开始长度为 n
的整数数组 nums
。
下标 i
处的 平均差 指的是 nums
中 前 i + 1
个元素平均值和 后 n - i - 1
个元素平均值的 绝对差 。两个平均值都需要 向下取整 到最近的整数。
请你返回产生 最小平均差 的下标。如果有多个下标最小平均差相等,请你返回 最小 的一个下标。
注意:
- 两个数的 绝对差 是两者差的绝对值。
-
n
个元素的平均值是n
个元素之 和 除以(整数除法)n
。 0
个元素的平均值视为0
。
示例 1:
输入:nums = [2,5,3,9,5,3]
输出:3
解释:
- 下标 0 处的平均差为:|2 / 1 - (5 + 3 + 9 + 5 + 3) / 5| = |2 / 1 - 25 / 5| = |2 - 5| = 3 。
- 下标 1 处的平均差为:|(2 + 5) / 2 - (3 + 9 + 5 + 3) / 4| = |7 / 2 - 20 / 4| = |3 - 5| = 2 。
- 下标 2 处的平均差为:|(2 + 5 + 3) / 3 - (9 + 5 + 3) / 3| = |10 / 3 - 17 / 3| = |3 - 5| = 2 。
- 下标 3 处的平均差为:|(2 + 5 + 3 + 9) / 4 - (5 + 3) / 2| = |19 / 4 - 8 / 2| = |4 - 4| = 0 。
- 下标 4 处的平均差为:|(2 + 5 + 3 + 9 + 5) / 5 - 3 / 1| = |24 / 5 - 3 / 1| = |4 - 3| = 1 。
- 下标 5 处的平均差为:|(2 + 5 + 3 + 9 + 5 + 3) / 6 - 0| = |27 / 6 - 0| = |4 - 0| = 4 。
下标 3 处的平均差为最小平均差,所以返回 3 。
示例 2:
输入:nums = [0]
输出:0
解释:
唯一的下标是 0 ,所以我们返回 0 。
下标 0 处的平均差为:|0 / 1 - 0| = |0 - 0| = 0 。
提示:
1 <= nums.length <= 105
0 <= nums[i] <= 105
Metadata
- Link: Minimum Average Difference
- Difficulty: Medium
- Tag:
You are given a 0-indexed integer array nums
of length n
.
The average difference of the index i
is the absolute difference between the average of the first i + 1
elements of nums
and the average of the last n - i - 1
elements. Both averages should be rounded down to the nearest integer.
Return the index with the minimum average difference. If there are multiple such indices, return the smallest one.
Note:
- The absolute difference of two numbers is the absolute value of their difference.
- The average of
n
elements is the sum of then
elements divided (integer division) byn
. - The average of
0
elements is considered to be0
.
Example 1:
Input: nums = [2,5,3,9,5,3]
Output: 3
Explanation:
- The average difference of index 0 is: |2 / 1 - (5 + 3 + 9 + 5 + 3) / 5| = |2 / 1 - 25 / 5| = |2 - 5| = 3.
- The average difference of index 1 is: |(2 + 5) / 2 - (3 + 9 + 5 + 3) / 4| = |7 / 2 - 20 / 4| = |3 - 5| = 2.
- The average difference of index 2 is: |(2 + 5 + 3) / 3 - (9 + 5 + 3) / 3| = |10 / 3 - 17 / 3| = |3 - 5| = 2.
- The average difference of index 3 is: |(2 + 5 + 3 + 9) / 4 - (5 + 3) / 2| = |19 / 4 - 8 / 2| = |4 - 4| = 0.
- The average difference of index 4 is: |(2 + 5 + 3 + 9 + 5) / 5 - 3 / 1| = |24 / 5 - 3 / 1| = |4 - 3| = 1.
- The average difference of index 5 is: |(2 + 5 + 3 + 9 + 5 + 3) / 6 - 0| = |27 / 6 - 0| = |4 - 0| = 4.
The average difference of index 3 is the minimum average difference so return 3.
Example 2:
Input: nums = [0]
Output: 0
Explanation:
The only index is 0 so return 0.
The average difference of index 0 is: |0 / 1 - 0| = |0 - 0| = 0.
Constraints:
1 <= nums.length <= 105
0 <= nums[i] <= 105
Solution
#include <bits/stdc++.h>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
#define endl "\n"
#define fi first
#define se second
#define all(x) begin(x), end(x)
#define rall rbegin(a), rend(a)
#define bitcnt(x) (__builtin_popcountll(x))
#define complete_unique(a) a.erase(unique(begin(a), end(a)), end(a))
#define mst(x, a) memset(x, a, sizeof(x))
#define MP make_pair
using ll = long long;
using ull = unsigned long long;
using db = double;
using ld = long double;
using VLL = std::vector<ll>;
using VI = std::vector<int>;
using PII = std::pair<int, int>;
using PLL = std::pair<ll, ll>;
using namespace __gnu_pbds;
using namespace std;
template <typename T>
using ordered_set = tree<T, null_type, less<T>, rb_tree_tag, tree_order_statistics_node_update>;
template <typename T, typename S>
inline bool chmax(T &a, const S &b) {
return a < b ? a = b, 1 : 0;
}
template <typename T, typename S>
inline bool chmin(T &a, const S &b) {
return a > b ? a = b, 1 : 0;
}
#ifdef LOCAL
#include <debug.hpp>
#else
#define dbg(...)
#endif
// head
class Solution {
public:
int minimumAverageDifference(vector<int> &nums) {
size_t n = nums.size();
auto pre = vector<ll>(n + 5, 0);
for (size_t i = 1; i <= n; i++) {
pre[i] = pre[i - 1] + nums[i - 1];
}
auto suffix = vector<ll>(n + 5, 0);
for (size_t i = n; i >= 1; i--) {
suffix[i] = suffix[i + 1] + nums[i - 1];
}
ll m = 0x3f3f3f3f;
int idx = -1;
for (size_t i = 1; i <= n; i++) {
ll a = static_cast<ll>(pre[i] / i);
ll b = 0;
if (n - i > 0) {
b = static_cast<ll>(suffix[i + 1] / (n - i));
}
ll cur = abs(a - b);
if (cur < m) {
m = cur;
idx = static_cast<int>(i - 1);
}
}
return idx;
}
};
#ifdef LOCAL
int main() {
return 0;
}
#endif
C
Statement
Metadata
- Link: 统计网格图中没有被保卫的格子数
- Difficulty: Medium
- Tag:
给你两个整数 m
和 n
表示一个下标从 0 开始的 m x n
网格图。同时给你两个二维整数数组 guards
和 walls
,其中 guards[i] = [rowi, coli]
且 walls[j] = [rowj, colj]
,分别表示第 i
个警卫和第 j
座墙所在的位置。
一个警卫能看到 4 个坐标轴方向(即东、南、西、北)的 所有 格子,除非他们被一座墙或者另外一个警卫 挡住 了视线。如果一个格子能被 至少 一个警卫看到,那么我们说这个格子被 保卫 了。
请你返回空格子中,有多少个格子是 没被保卫 的。
示例 1:
输入:m = 4, n = 6, guards = [[0,0],[1,1],[2,3]], walls = [[0,1],[2,2],[1,4]]
输出:7
解释:上图中,被保卫和没有被保卫的格子分别用红色和绿色表示。
总共有 7 个没有被保卫的格子,所以我们返回 7 。
示例 2:
输入:m = 3, n = 3, guards = [[1,1]], walls = [[0,1],[1,0],[2,1],[1,2]]
输出:4
解释:上图中,没有被保卫的格子用绿色表示。
总共有 4 个没有被保卫的格子,所以我们返回 4 。
提示:
1 <= m, n <= 105
2 <= m * n <= 105
1 <= guards.length, walls.length <= 5 * 104
2 <= guards.length + walls.length <= m * n
guards[i].length == walls[j].length == 2
0 <= rowi, rowj < m
0 <= coli, colj < n
guards
和walls
中所有位置 互不相同 。
Metadata
- Link: Count Unguarded Cells in the Grid
- Difficulty: Medium
- Tag:
You are given two integers m
and n
representing a 0-indexed m x n
grid. You are also given two 2D integer arrays guards
and walls
where guards[i] = [rowi, coli]
and walls[j] = [rowj, colj]
represent the positions of the ith
guard and jth
wall respectively.
A guard can see every cell in the four cardinal directions (north, east, south, or west) starting from their position unless obstructed by a wall or another guard. A cell is guarded if there is at least one guard that can see it.
Return the number of unoccupied cells that are not guarded.
Example 1:
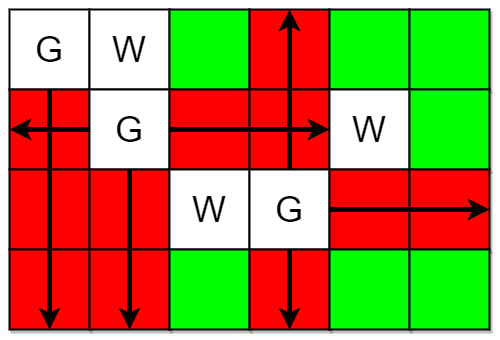
Input: m = 4, n = 6, guards = [[0,0],[1,1],[2,3]], walls = [[0,1],[2,2],[1,4]]
Output: 7
Explanation: The guarded and unguarded cells are shown in red and green respectively in the above diagram.
There are a total of 7 unguarded cells, so we return 7.
Example 2:
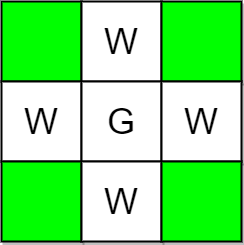
Input: m = 3, n = 3, guards = [[1,1]], walls = [[0,1],[1,0],[2,1],[1,2]]
Output: 4
Explanation: The unguarded cells are shown in green in the above diagram.
There are a total of 4 unguarded cells, so we return 4.
Constraints:
1 <= m, n <= 105
2 <= m * n <= 105
1 <= guards.length, walls.length <= 5 * 104
2 <= guards.length + walls.length <= m * n
guards[i].length == walls[j].length == 2
0 <= rowi, rowj < m
0 <= coli, colj < n
- All the positions in
guards
andwalls
are unique.
Solution
#include <bits/stdc++.h>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
#include <vector>
#define endl "\n"
#define fi first
#define se second
#define all(x) begin(x), end(x)
#define rall rbegin(a), rend(a)
#define bitcnt(x) (__builtin_popcountll(x))
#define complete_unique(a) a.erase(unique(begin(a), end(a)), end(a))
#define mst(x, a) memset(x, a, sizeof(x))
#define MP make_pair
using ll = long long;
using ull = unsigned long long;
using db = double;
using ld = long double;
using VLL = std::vector<ll>;
using VI = std::vector<int>;
using PII = std::pair<int, int>;
using PLL = std::pair<ll, ll>;
using namespace __gnu_pbds;
using namespace std;
template <typename T>
using ordered_set = tree<T, null_type, less<T>, rb_tree_tag, tree_order_statistics_node_update>;
template <typename T, typename S>
inline bool chmax(T &a, const S &b) {
return a < b ? a = b, 1 : 0;
}
template <typename T, typename S>
inline bool chmin(T &a, const S &b) {
return a > b ? a = b, 1 : 0;
}
#ifdef LOCAL
#include <debug.hpp>
#else
#define dbg(...)
#endif
// head
class Solution {
public:
int countUnguarded(int n, int m, vector<vector<int>> &guards, vector<vector<int>> &walls) {
auto vis = vector<vector<int>>(n + 1, vector<int>(m + 1, 0));
for (const auto &g : guards) {
vis[g[0]][g[1]] = 2;
}
for (const auto &w : walls) {
vis[w[0]][w[1]] = 3;
}
for (int i = 0; i < n; i++) {
for (int j = 0, cur = -1; j < m; j++) {
if (vis[i][j] > 1) {
cur = vis[i][j];
} else if (cur == 2) {
vis[i][j] = 1;
}
}
for (int j = m - 1, cur = -1; j >= 0; j--) {
if (vis[i][j] > 1) {
cur = vis[i][j];
} else if (cur == 2) {
vis[i][j] = 1;
}
}
}
for (int j = 0; j < m; j++) {
for (int i = 0, cur = -1; i < n; i++) {
if (vis[i][j] > 1) {
cur = vis[i][j];
} else if (cur == 2) {
vis[i][j] = 1;
}
}
for (int i = n - 1, cur = -1; i >= 0; i--) {
if (vis[i][j] > 1) {
cur = vis[i][j];
} else if (cur == 2) {
vis[i][j] = 1;
}
}
}
int res = 0;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (vis[i][j] == 0) {
++res;
}
}
}
return res;
}
};
#ifdef LOCAL
int main() {
return 0;
}
#endif
D
Statement
Metadata
- Link: 逃离火灾
- Difficulty: Hard
- Tag:
给你一个下标从 0 开始大小为 m x n
的二维整数数组 grid
,它表示一个网格图。每个格子为下面 3 个值之一:
0
表示草地。1
表示着火的格子。2
表示一座墙,你跟火都不能通过这个格子。
一开始你在最左上角的格子 (0, 0)
,你想要到达最右下角的安全屋格子 (m - 1, n - 1)
。每一分钟,你可以移动到 相邻 的草地格子。每次你移动 之后 ,着火的格子会扩散到所有不是墙的 相邻 格子。
请你返回你在初始位置可以停留的 最多 分钟数,且停留完这段时间后你还能安全到达安全屋。如果无法实现,请你返回 -1
。如果不管你在初始位置停留多久,你 总是 能到达安全屋,请你返回 109
。
注意,如果你到达安全屋后,火马上到了安全屋,这视为你能够安全到达安全屋。
如果两个格子有共同边,那么它们为 相邻 格子。
示例 1:
输入:grid = [[0,2,0,0,0,0,0],[0,0,0,2,2,1,0],[0,2,0,0,1,2,0],[0,0,2,2,2,0,2],[0,0,0,0,0,0,0]]
输出:3
解释:上图展示了你在初始位置停留 3 分钟后的情形。
你仍然可以安全到达安全屋。
停留超过 3 分钟会让你无法安全到达安全屋。
示例 2:
输入:grid = [[0,0,0,0],[0,1,2,0],[0,2,0,0]]
输出:-1
解释:上图展示了你马上开始朝安全屋移动的情形。
火会蔓延到你可以移动的所有格子,所以无法安全到达安全屋。
所以返回 -1 。
示例 3:
输入:grid = [[0,0,0],[2,2,0],[1,2,0]]
输出:1000000000
解释:上图展示了初始网格图。
注意,由于火被墙围了起来,所以无论如何你都能安全到达安全屋。
所以返回 109 。
提示:
m == grid.length
n == grid[i].length
2 <= m, n <= 300
4 <= m * n <= 2 * 104
grid[i][j]
是0
,1
或者2
。grid[0][0] == grid[m - 1][n - 1] == 0
Metadata
- Link: Escape the Spreading Fire
- Difficulty: Hard
- Tag:
You are given a 0-indexed 2D integer array grid
of size m x n
which represents a field. Each cell has one of three values:
0
represents grass,1
represents fire,2
represents a wall that you and fire cannot pass through.
You are situated in the top-left cell, (0, 0)
, and you want to travel to the safehouse at the bottom-right cell, (m - 1, n - 1)
. Every minute, you may move to an adjacent grass cell. After your move, every fire cell will spread to all adjacent cells that are not walls.
Return the maximum number of minutes that you can stay in your initial position before moving while still safely reaching the safehouse. If this is impossible, return -1
. If you can always reach the safehouse regardless of the minutes stayed, return 109
.
Note that even if the fire spreads to the safehouse immediately after you have reached it, it will be counted as safely reaching the safehouse.
A cell is adjacent to another cell if the former is directly north, east, south, or west of the latter (i.e., their sides are touching).
Example 1:
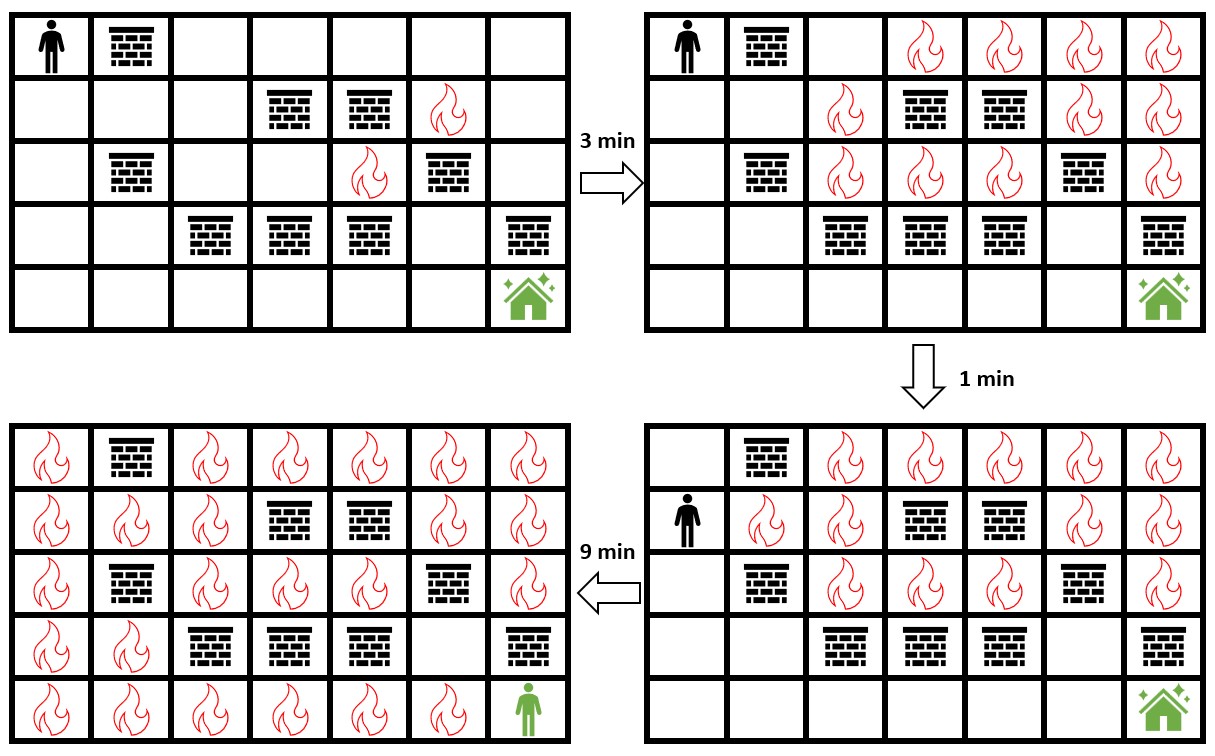
Input: grid = [[0,2,0,0,0,0,0],[0,0,0,2,2,1,0],[0,2,0,0,1,2,0],[0,0,2,2,2,0,2],[0,0,0,0,0,0,0]]
Output: 3
Explanation: The figure above shows the scenario where you stay in the initial position for 3 minutes.
You will still be able to safely reach the safehouse.
Staying for more than 3 minutes will not allow you to safely reach the safehouse.
Example 2:
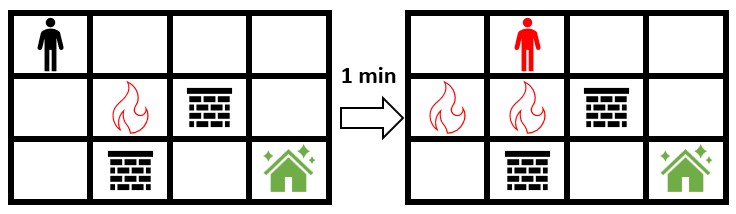
Input: grid = [[0,0,0,0],[0,1,2,0],[0,2,0,0]]
Output: -1
Explanation: The figure above shows the scenario where you immediately move towards the safehouse.
Fire will spread to any cell you move towards and it is impossible to safely reach the safehouse.
Thus, -1 is returned.
Example 3:
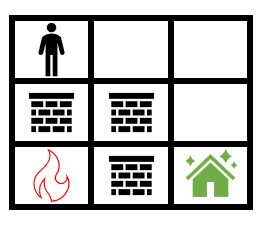
Input: grid = [[0,0,0],[2,2,0],[1,2,0]]
Output: 1000000000
Explanation: The figure above shows the initial grid.
Notice that the fire is contained by walls and you will always be able to safely reach the safehouse.
Thus, 109 is returned.
Constraints:
m == grid.length
n == grid[i].length
2 <= m, n <= 300
4 <= m * n <= 2 * 104
grid[i][j]
is either0
,1
, or2
.grid[0][0] == grid[m - 1][n - 1] == 0
Solution
#include <bits/stdc++.h>
#include <ext/pb_ds/assoc_container.hpp>
#include <ext/pb_ds/tree_policy.hpp>
#include <vector>
#define endl "\n"
#define fi first
#define se second
#define all(x) begin(x), end(x)
#define rall rbegin(a), rend(a)
#define bitcnt(x) (__builtin_popcountll(x))
#define complete_unique(a) a.erase(unique(begin(a), end(a)), end(a))
#define mst(x, a) memset(x, a, sizeof(x))
#define MP make_pair
using ll = long long;
using ull = unsigned long long;
using db = double;
using ld = long double;
using VLL = std::vector<ll>;
using VI = std::vector<int>;
using PII = std::pair<int, int>;
using PLL = std::pair<ll, ll>;
using namespace __gnu_pbds;
using namespace std;
template <typename T>
using ordered_set = tree<T, null_type, less<T>, rb_tree_tag, tree_order_statistics_node_update>;
template <typename T, typename S>
inline bool chmax(T &a, const S &b) {
return a < b ? a = b, 1 : 0;
}
template <typename T, typename S>
inline bool chmin(T &a, const S &b) {
return a > b ? a = b, 1 : 0;
}
#ifdef LOCAL
#include <debug.hpp>
#else
#define dbg(...)
#endif
// head
struct node {
int x, y, t;
};
int mv[][2] = {
{0, 1},
{0, -1},
{1, 0},
{-1, 0},
};
class Solution {
public:
int maximumMinutes(vector<vector<int>> &grid) {
int n = static_cast<int>(grid.size());
int m = static_cast<int>(grid[0].size());
auto ok = [&](int x, int y) {
if (x < 0 || x >= n || y < 0 || y >= m) {
return false;
}
return true;
};
auto check = [&](int x) -> bool {
queue<node> f, p;
auto g = vector<vector<int>>(grid);
auto visf = vector<vector<int>>(n + 1, vector<int>(m + 1, 0));
auto visp = vector<vector<int>>(n + 1, vector<int>(m + 1, 0));
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (g[i][j] == 1) {
f.push({
.x = i,
.y = j,
.t = 0,
});
visf[i][j] = 1;
}
}
}
auto inc = [&](int tt) {
while (!f.empty()) {
auto ff = f.front();
int x = ff.x;
int y = ff.y;
int t = ff.t;
if (t >= tt) {
break;
}
f.pop();
g[x][y] = 1;
for (int i = 0; i < 4; i++) {
int nx = x + mv[i][0];
int ny = y + mv[i][1];
if (ok(nx, ny) && visf[nx][ny] == 0 && g[nx][ny] == 0) {
visf[nx][ny] = 1;
f.push({
.x = nx,
.y = ny,
.t = t + 1,
});
}
}
}
};
inc(x);
if (g[0][0] != 0) {
return false;
}
p.push({
.x = 0,
.y = 0,
.t = x,
});
visp[0][0] = 1;
while (!p.empty()) {
auto pp = p.front();
p.pop();
int x = pp.x;
int y = pp.y;
int t = pp.t;
inc(t + 1);
if (x == n - 1 && y == m - 1) {
return true;
}
if (g[x][y] == 1) {
continue;
}
for (int i = 0; i < 4; i++) {
int nx = x + mv[i][0];
int ny = y + mv[i][1];
if (ok(nx, ny) && visp[nx][ny] == 0 && g[nx][ny] == 0) {
visp[nx][ny] = 1;
p.push({
.x = nx,
.y = ny,
.t = t + 1,
});
}
}
}
return false;
};
int l = 0, r = 1e9, res = -1;
while (r - l >= 0) {
int mid = (l + r) >> 1;
if (check(mid)) {
l = mid + 1;
res = mid;
} else {
r = mid - 1;
}
}
return res;
}
};
#ifdef LOCAL
int main() {
return 0;
}
#endif